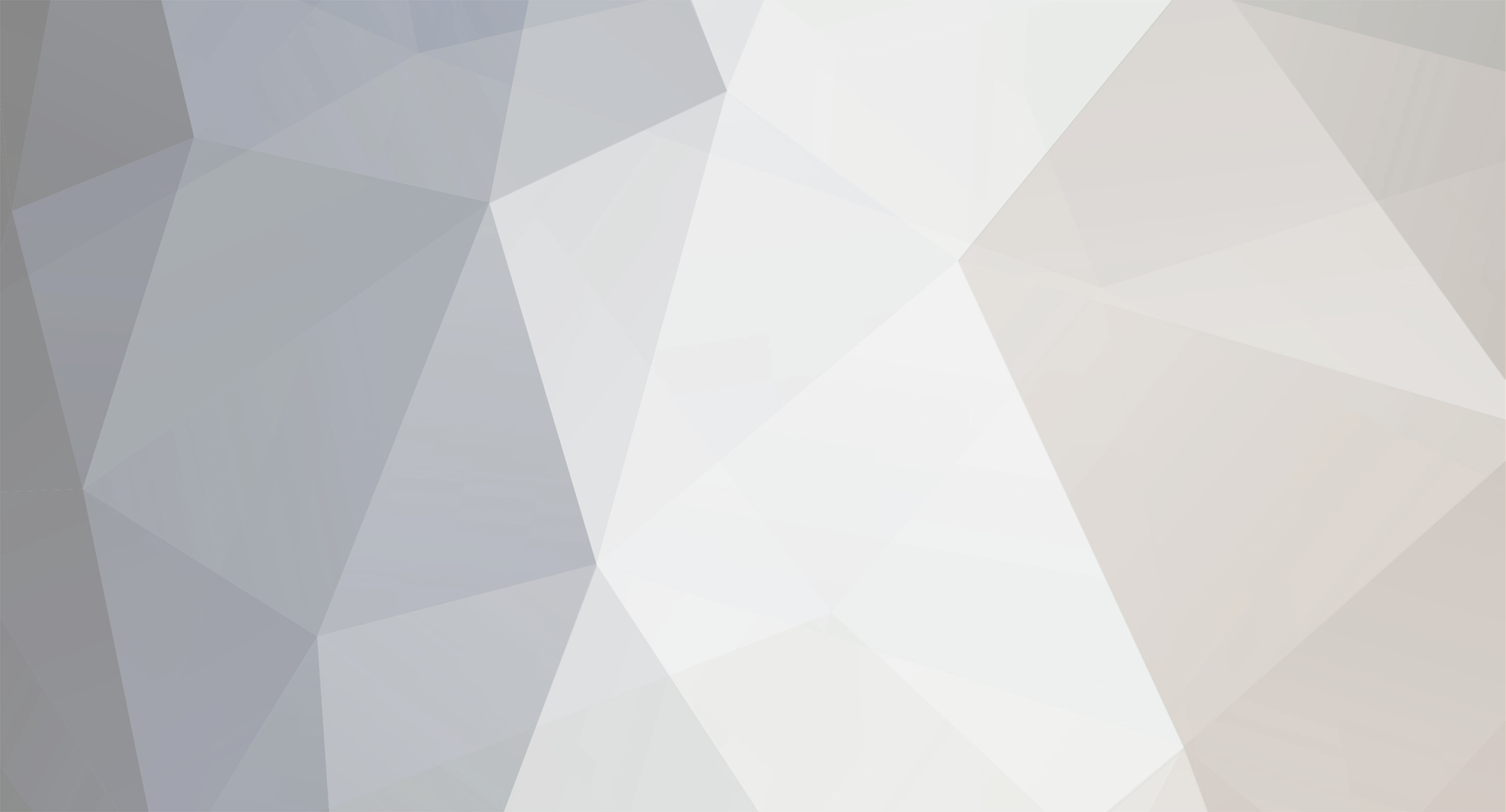
pilo
Frequent Writer-
Posts
1,093 -
Joined
-
Last visited
-
Days Won
4
Content Type
Profiles
Forums
Blogs
Gallery
Everything posted by pilo
-
Hi! I'm not sure if I understand your problem correctly, but it seems like a general code design problem? If I understand well, you have things that should happen when there's a Midi notify or a button press? A simple way to handle this is simply putting the code that does something outside the MIOS call back : void doSomething(u32 value) { // put your code here } void APP_MIDI_NotifyPackage(mios32void APP_MIDI_NotifyPackage(mios32_midi_port_t port, mios32_midi_package_t midi_package){ if(/*condition*/) { doSomething(1); // or doSomething(0) } } #define doSomethingButton 4 /* for example */ void APP_DIN_NotifyToggle(u32 pin, u32 pin_value) { if(pin == doSomethingButton) { doSomething(pin_value); } } Now for the problem with flag (it's a "common" problem, I still see a lots of code with flags every where, and when you want to modify to do something, it does everything but not what you want it to do!). There are several way to handle this. A good one (but it can be tricky to implement sometimes) is the state pattern. Instead of having flags and then testing flags, you replace those by on or more states. States can be function in C, and then you keep a pointer to the current state function, which will do what it should when the app is in this state. I don't have a good example to show this, but if you want to post your code, I can take time to look at it if you want.
- 3 replies
-
- LPC17force function
- triggering events
-
(and 2 more)
Tagged with:
-
Thank you ;) When i said it might be a hardware issue, I was thinking about something like wiring issue. I fried my testing psu (for the analog +5/-5v) yesterday while working on this, I might have made a modification to the code that prevent it from working after (I wrote the value to dB code after my PSU made some smoke). Also it would be a shame both your PGA chips are broken. I think before ordering new ones there are other things to check ;) First, I use lpc17 core, I don't think it should make a big difference, but maybe it is. Then how did you wire your power supply? I use the +5v from the core for the digital side of the PGA, and another external PSU for the analog side (I build a new one today, not finished yet). Both ground (analog and digital) are connected. Mute and ZCEN are connected to the +5v from the core. Of course analog and digital +5v ARE NOT connected together. Double check your wiring, there might be an error somewhere. No sound at all when you run the application? (Without turning any encoder)
-
Here's my test app, it should work now (unless you have an hardware issue). /* * MIOS32 TI PGA2310/2311/4311 test app * * ========================================================================== * * Copyright © 2015 pilo () * Licensed for personal non-commercial use only. * All other rights reserved. * * ========================================================================== */ ///////////////////////////////////////////////////////////////////////////// // Include files ///////////////////////////////////////////////////////////////////////////// #include <mios32.h> #include "app.h" // warning, buffer should be at least 9 byte long! void PGAGainTodB(char *buffer, u8 gain) { if(gain == 0) { sprintf(buffer, "mute"); return; } // else int _dB = 315 - (1275 - gain * 5); int dB = fabs(_dB / 10); int mod = fabs(_dB % 10); if(mod == 0) sprintf(buffer, "%ddB", _dB / 10); else sprintf(buffer, "%s%d%s%ddB", _dB < 0 ? "-" : "", // sign dB, mod != 0 ? "." : "", mod != 0 ? mod : ""); } void SetPGAGain(u8 leftGain, u8 rightGain) { MIOS32_SPI_RC_PinSet (2,0,0); MIOS32_SPI_TransferByte(2, rightGain); // right MIOS32_SPI_TransferByte(2, leftGain); // left MIOS32_SPI_TransferByte(2, rightGain); // 3 (for PGA4311) MIOS32_SPI_TransferByte(2, leftGain); // 4 MIOS32_SPI_RC_PinSet (2,0,1); //MIOS32_MIDI_SendDebugMessage("SetPGAGain : %d %d\n", leftGain, rightGain); } u8 volumeEncPos = 192; // 0dB #define NUM_ENCODERS 64 ///////////////////////////////////////////////////////////////////////////// // This hook is called after startup to initialize the application ///////////////////////////////////////////////////////////////////////////// void APP_Init(void) { // initialize all LEDs MIOS32_BOARD_LED_Init(0xffffffff); // initialize SPI IO pins and protocol MIOS32_SPI_IO_Init(2, MIOS32_SPI_PIN_DRIVER_WEAK); MIOS32_SPI_TransferModeInit(2, MIOS32_SPI_MODE_CLK0_PHASE0, MIOS32_SPI_PRESCALER_16); // ca. 5 MBit // initialize rotary encoders of the same type (DETENTED2) int enc; for(enc=0; enc<NUM_ENCODERS; ++enc) { u8 pin_sr = (enc >> 2) + 1; // each DIN SR has 4 encoders connected u8 pin_pos = (enc & 0x3) << 1; // Pin position of first ENC channel: either 0, 2, 4 or 6 mios32_enc_config_t enc_config = MIOS32_ENC_ConfigGet(enc); enc_config.cfg.type = DETENTED2; // see mios32_enc.h for available types enc_config.cfg.sr = pin_sr; enc_config.cfg.pos = pin_pos; #if 1 // normal speed, incrementer either 1 or -1 enc_config.cfg.speed = NORMAL; enc_config.cfg.speed_par = 0; #else // higher incrementer values on fast movements enc_config.cfg.speed = FAST; enc_config.cfg.speed_par = 2; #endif MIOS32_ENC_ConfigSet(enc, enc_config); } } ///////////////////////////////////////////////////////////////////////////// // This task is running endless in background ///////////////////////////////////////////////////////////////////////////// void APP_Background(void) { MIOS32_MIDI_SendDebugMessage("Started PGA test app\n"); SetPGAGain(volumeEncPos, volumeEncPos); while(1) { } } ///////////////////////////////////////////////////////////////////////////// // This hook is called each mS from the main task which also handles DIN, ENC // and AIN events. You could add more jobs here, but they shouldn't consume // more than 300 uS to ensure the responsiveness of buttons, encoders, pots. // Alternatively you could create a dedicated task for application specific // jobs as explained in $MIOS32_PATH/apps/tutorials/006_rtos_tasks ///////////////////////////////////////////////////////////////////////////// void APP_Tick(void) { // PWM modulate the status LED (this is a sign of life) u32 timestamp = MIOS32_TIMESTAMP_Get(); MIOS32_BOARD_LED_Set(1, (timestamp % 20) <= ((timestamp / 100) % 10)); } ///////////////////////////////////////////////////////////////////////////// // This hook is called each mS from the MIDI task which checks for incoming // MIDI events. You could add more MIDI related jobs here, but they shouldn't // consume more than 300 uS to ensure the responsiveness of incoming MIDI. ///////////////////////////////////////////////////////////////////////////// void APP_MIDI_Tick(void) { } ///////////////////////////////////////////////////////////////////////////// // This hook is called when a MIDI package has been received ///////////////////////////////////////////////////////////////////////////// void APP_MIDI_NotifyPackage(mios32_midi_port_t port, mios32_midi_package_t midi_package) { } ///////////////////////////////////////////////////////////////////////////// // This hook is called before the shift register chain is scanned ///////////////////////////////////////////////////////////////////////////// void APP_SRIO_ServicePrepare(void) { } ///////////////////////////////////////////////////////////////////////////// // This hook is called after the shift register chain has been scanned ///////////////////////////////////////////////////////////////////////////// void APP_SRIO_ServiceFinish(void) { } ///////////////////////////////////////////////////////////////////////////// // This hook is called when a button has been toggled // pin_value is 1 when button released, and 0 when button pressed ///////////////////////////////////////////////////////////////////////////// void APP_DIN_NotifyToggle(u32 pin, u32 pin_value) { } ///////////////////////////////////////////////////////////////////////////// // This hook is called when an encoder has been moved // incrementer is positive when encoder has been turned clockwise, else // it is negative ///////////////////////////////////////////////////////////////////////////// void APP_ENC_NotifyChange(u32 encoder, s32 incrementer) { int value = volumeEncPos + incrementer; // clamp the value if(value < 0) value = 0; if(value > 255) value = 255; // set the gain volumeEncPos = value; SetPGAGain(volumeEncPos, volumeEncPos); // print gain in dB char dbBuffer[9]; PGAGainTodB(dbBuffer, volumeEncPos); MIOS32_MIDI_SendDebugMessage("%s\n", dbBuffer); } ///////////////////////////////////////////////////////////////////////////// // This hook is called when a pot has been moved ///////////////////////////////////////////////////////////////////////////// void APP_AIN_NotifyChange(u32 pin, u32 pin_value) { }
-
Hi, Ok good news, I got it working :smile: I use PGA4311 (SMD IC), and my mute pin, after living 10 years in a junk box was broken (I made an error and connect it to GND instead on +5V on my original PCB, and then use a wire to correct this... but the pin didn't handle it over the years). So the modification I made is in APP_init: MIOS32_SPI_IO_Init(2, MIOS32_SPI_PIN_DRIVER_WEAK); MIOS32_SPI_TransferModeInit(2, MIOS32_SPI_MODE_CLK0_PHASE0, MIOS32_SPI_PRESCALER_16); // ca. 5 MBit I don't really understand how this and prescaler work, but with those settings I can set the gain. I'll add encoder support for volume change and post the source code.
-
Hi! Do you setup the SPI intervace in APP_init() ? (I think yes, but it's always good to check everything ;) // initialize SPI IO pins and protocol MIOS32_SPI_IO_Init(2, MIOS32_SPI_PIN_DRIVER_STRONG); MIOS32_SPI_TransferModeInit(2, MIOS32_SPI_MODE_CLK0_PHASE0, MIOS32_SPI_PRESCALER_16); // ca. 5 MBit I don't understand why it doesn't work (and unfortunately I don't have my old PGA pcb here with me). I think the command is not working because there's no command handling callback ;) (you can setup one, but you have to parse it etc). I had the idea few month ago to make a preamp with the PGA, but it was a few month ago and I haven't done anything yet... :)
-
Hi mwpost! Unfortunately I didn't had the time this week end to test my old pga pcb with MIOS32 (I have an LPC17 board, not STM32F4, but it shouldn't be a big difference for this). What you could do, is to try to set the gain without the encoder first (comment or remove the code setting the gain in the encoder callback). just add this function in your c file : void SetPGAGain(u8 leftGain, u8 rightGain) { MIOS32_SPI_RC_PinSet (2,0,0); MIOS32_SPI_TransferByte(2, mios32_dout_reverse_tab[rightGain]); // right MIOS32_SPI_TransferByte(2, mios32_dout_reverse_tab[leftGain]); // left MIOS32_SPI_RC_PinSet (2,0,1); } and for example in APP_Background void APP_Background(void) { SetPGAGain(0, 0); while (1) { } } When you start the application, you should hear no sound at all (0 is the special value for MUTE). Then try for example SetPGAGain(32, 32); etc and then with other values, and see how it behave. It might help to understand what is going on by just changing the value once. There's no "useless hobby" :smile: I was very excited by the PGA at that time, I ordered some, made a quick board, wrote the code for MIOS... and when it worked, I though "Cool, I need to put it in a very cool project!", and I haven't touch it since... (like so many other "project" I started)
-
Hi mwpost! I wrote PGA driver code for pic18f years ago (looking at the source code, it should make 10 years...), which was later improved by Lyle. I remember having fun writing the display part in asm :smile: Anyway, I'll try to make it work with MIOS32. I think you're very close to have it working, actually I don't see any problem with your code (if you does the modification correctly). According to your schematic only the left part of the PGA is tested, and that's the 2nd byte send to the PGA : void APP_ENC_NotifyChange(u32 encoder, s32 incrementer) { // toggle Status LED on each AIN value change MIOS32_BOARD_LED_Set(0x0001, ~MIOS32_BOARD_LED_Get()); // increment to virtual position and ensure that the value is in range 0..127 int value = enc_virtual_pos[encoder] + incrementer; // clamp the value if( value < 0 ) value = 0; else if( value > 255 ) value = 255; // store enc_virtual_pos[encoder] = value; MIOS32_MIDI_SendCC(UART0, Chn3, 53, value); MIOS32_MIDI_SendCC(USB0, Chn3, 53, value); u8 Sendbyte = mios32_dout_reverse_tab[value]; // MSB first MIOS32_MIDI_SendDebugMessage("MIOS32_SPI_RC_PinSet to 0: %04u", MIOS32_SPI_RC_PinSet (2,1,0)); MIOS32_MIDI_SendDebugMessage("MIOS32_SPI_TransferByte R %04u: %04u", Sendbyte, MIOS32_SPI_TransferByte(2, Sendbyte)); // right channel gain MIOS32_MIDI_SendDebugMessage("MIOS32_SPI_TransferByte L: %04u: %04u", Sendbyte, MIOS32_SPI_TransferByte(2, Sendbyte)); // left channel gain MIOS32_MIDI_SendDebugMessage("MIOS32_SPI_RC_PinSet to 1: %04u", MIOS32_SPI_RC_PinSet (2,1,1)); MIOS32_LCD_CursorSet(10, 1); MIOS32_LCD_PrintFormattedString("%04u", Sendbyte); } If you daisy chained PGA (or use PGA4311) you should have as many MIOS32_SPI_TransferByte as channels (so you need 2 for one PGA2311, 4 for 2 chained PGA2311, 4 for one PGA4311, 6 for one PGA4311 and one PGA2311, etc). And I wouldn't expect any value returned by MIOS32_SPI_TransferByte?
-
Linux - Mios8 - Toochain - make - problems
pilo replied to Phatline's topic in MIDIbox Tools & MIOS Studio
I just removed lastest sdcc version and installed sdcc from the link provided by TK, and everything works as expected now :) (without any modification). -
Linux - Mios8 - Toochain - make - problems
pilo replied to Phatline's topic in MIDIbox Tools & MIOS Studio
This is not a problem with sdcc install, because here what the makefile is trying to execute : /bin/share/sdcc /home/crimic/mios/bin/mios-gpasm -c -p p18f452 -I./src -I /home/crimic/mios/include/asm -I /home/crimic/mios/include/share -I /home/crimic/mios/modules/debug_msg -I /home/crimic/mios/modules/app_lcd/dummy -DDEBUG_MODE=0 -DSTACK_HEAD=0x37f -DSTACK_IRQ_HEAD=0x33f -I /home/crimic/mios/modules/mios_wrapper /home/crimic/mios/modules/mios_wrapper/mios_wrapper.asm -o _output/mios_wrapper.o I don't know why sdcc and mios-gpasm are mixed here, but it should be only : /home/crimic/mios/bin/mios-gpasm -c -p p18f452 -I./src -I /home/crimic/mios/include/asm -I /home/crimic/mios/include/share -I /home/crimic/mios/modules/debug_msg -I /home/crimic/mios/modules/app_lcd/dummy -DDEBUG_MODE=0 -DSTACK_HEAD=0x37f -DSTACK_IRQ_HEAD=0x33f -I /home/crimic/mios/modules/mios_wrapper /home/crimic/mios/modules/mios_wrapper/mios_wrapper.asm -o _output/mios_wrapper.o edit: Maybe it's the path to bin that is not correct? echo $MIOS_BIN_PATH should tell you "/home/crimic/mios/bin" and it seems it's set on "/bin/share/sdcc /home/crimic/mios/bin" -
Linux - Mios8 - Toochain - make - problems
pilo replied to Phatline's topic in MIDIbox Tools & MIOS Studio
Hi! I just install it to try it on my linux computer (Slackware, but it shouldn't be too different from ubuntu). I had the same error as you get. You can try to fix by editing mios-sdcc (mios/bin/mios-sdcc) and add "--use-non-free" as sdcc parameter My sdcc-mios looks like that now : # $Id: mios-sdcc 444 2008-08-14 21:26:28Z tk $ # # MIOS specific wrapper for sdcc # # Executes sdcc # Uses "mios-gpasm -fixasm" wrapper to assemble the generated code # # Thorsten Klose (2008-02-03) # # SYNTAX: mios-sdcc <sdcc-arguments> # if [ -z "${MIOS_BIN_PATH:-}" ]; then echo "ERROR $0: MIOS_BIN_PATH variable not set!" exit 1 fi sdcc --use-non-free --asm="${MIOS_SHELL} ${MIOS_BIN_PATH}/mios-gpasm -fixasm" $@ I still have another error (according to your log you don't have it) pilo@linux-pilo:~/src/mios/mios/trunk/apps/examples/lcd7/dog/c$ make rm -rf _output/* rm -rf _output rm -rf *.cod *.map *.lst rm -rf *.hex mkdir -p _output /bin/bash /home/pilo/src/mios/mios/trunk/bin/mios-gpasm -c -p p18f452 -I./src -I /home/pilo/src/mios/mios/trunk/include/asm -I /home/pilo/src/mios/mios/trunk/include/share -I /home/pilo/src/mios/mios/trunk/modules/app_lcd/dog -DDEBUG_MODE=0 -DSTACK_HEAD=0x37f -DSTACK_IRQ_HEAD=0x33f -I /home/pilo/src/mios/mios/trunk/modules/mios_wrapper /home/pilo/src/mios/mios/trunk/modules/mios_wrapper/mios_wrapper.asm -o _output/mios_wrapper.o /bin/bash /home/pilo/src/mios/mios/trunk/bin/mios-gpasm -c -p p18f452 -I./src -I /home/pilo/src/mios/mios/trunk/include/asm -I /home/pilo/src/mios/mios/trunk/include/share -I /home/pilo/src/mios/mios/trunk/modules/app_lcd/dog -DDEBUG_MODE=0 /home/pilo/src/mios/mios/trunk/modules/app_lcd/dog/app_lcd.asm -o _output/app_lcd.o /bin/bash /home/pilo/src/mios/mios/trunk/bin/mios-sdcc -c -mpic16 -p18f452 --fommit-frame-pointer --optimize-goto --optimize-cmp --disable-warning 85 --obanksel=2 -I./src -I /home/pilo/src/mios/mios/trunk/include/c -I /home/pilo/src/mios/mios/trunk/include/share -DDEBUG_MODE=0 main.c -o _output/main.o at 1: warning 118: option '--fommit-frame-pointer-ÿ' no longer supported 'use --fomit-frame-pointer instead' at 1: warning 117: unknown compiler option '--optimize-goto' ignored /home/pilo/src/mios/mios/trunk/include/c/cmios.h:250: syntax error: token -> 'char' ; column 43 make: *** [_output/main.o] Error 1 (might be a compile flag I didn't set when I compile sdcc from source) To answer you question: 1. Yes it seems it's correct 2. You can also grab the latest code from the svn (svn checkout svn://svnmios.midibox.org/mios if you have svn installed). Then you'll find everything in mios/trunk/) I'll try to fix my setup, and I hope you'll manage to make yours working! -
Hi! I had the LPC17 core PCB for a long time, but ordered the LPC1769 only last week. Yesterday morning when I wanted to solder the header I was surprised when I noticed (before soldering) that they don't match the one on the PCB... After reading that post, I decided to solder them, remove the black plastic holder, and bend the pin to match the already soldered(about 1 year ago...) female connector on the PCB (The header on LPC1769 were soldered not "fully inserted", so I have enough room on each pin to bend them 2 times). Here's some pictures :
-
Ok :) En effet c'est pas n'importe quel console!!! Si il y a un système d'automation, c'est que les faders (ils sont motorisés ou pas du coup?) possèdent une piste servo (linéaire) en plus de la piste audio, donc il suffit d'utiliser celle ci. C'est le moyen le plus simple de récupérer la position. Et si ils sont motorisés tu peux peut etre aussi les controller avec le module MF, si le driver et les moteurs sont compatible.
-
C'est quoi comme modèle de table? Il faut le schema pour ce qu'il est possible de faire. Dans le cas de la midibox la lecture d'un fader ce fait simplement avec un adc (dans le pic ou l'arm suivant le core choisit). Pour le core 8bits : http://www.ucapps.de/mbhp_core.html Et le 32bits : http://www.ucapps.de/mbhp_core_lpc17.html
-
Salut! Alors tu ne pourras pas, ou très difficilement (comprendre couteux et pas forcément très pratique) ajouter ce genre de fonctionnalité sur une table de mixage analogique. Les fader utilisés pour l'audio utilise une piste a variation log, alors que pour une midibox il te faut des faders a variation linéaire. Tu dois pouvoir trouver des fader proposant 2 pistes comme ca, (comme les faders motorisé Alps), mais ca risque de couter relativement cher (et il faut que tu puisse les monter à la place des fader originaux qui sont dans ta table, ce qui n'est pas forcément possible). Et surtout, si tu veux baisser le volume d'une voie de ta table, cela va aussi baissé la voie controller dans le DAW, et vice versa. Un moyen plus simple serait de creer un controller séparé et dédié, avec 32 faders (et eventuelement d'autre switch, encoder, etc).
-
MIDIbox FM V2.0 Prototype: Improperly Configured
pilo commented on Sauraen's gallery image in MIDIbox Gallery
-
You could use a simple output for the AL1201 (look in the datasheet) if you don't need a very good "sound" (I guess for cv it should be fine).
-
I put brd ans sch file in a zip and it works :) al1201 dac.zip
-
Ouais en france aussi on a la douane qui peut attraper un colis ici ou la. Théoriquement justement, quand on commande sur le site de smash via le liens que j'ai donné, ca devrait passé... mais y'a rien de sur (j'ai commandé plusieurs fois, et jusqu'a maintenant aucun soucis). Ok pour l'insoleuse :) Un collègue viens de me dire que je pourrais simplement collé les supports avec un pistolet a colle... a essayer donc (mais comme je les veux au dessus , donc la tete en bas, j'aimerai etre sur qu'ils ne tombent pas! lol).
-
Salut Alchemist! Alors pour les circuits imprimés, l'usage d'une insoleuse est toujours d'actualité ;) Il exist cependant des méthodes alternatives. Pour le double face, il faut etre méticuleux, avec une insoleuse ca devrait être le plus facile (tu as une insoleuse simple ou double face?). Pour graver, j'ai une graveuse "economique", avec chauffage et oxygénation d'aquarium et je ne l'utilise plus... je grave maintenant dans un petit bac en plastique, que je place dans un peu d'eau chaude dans un lavabo (ca marche pas si mal), un peu comme toi donc je pense :) Concernant la méthode alternative, c'est d'utiliser le transfert de toner : il existe des papiers spécialisés : press'n peel blue, assez cher, mais il existe aussi des papier "chinois" très economique, ou encore certain le font avec du papier glacé de magazine. Le principe est simple, tu imprime (avec une imprimante lazer) sur ce papier, et ensuite avec un fer a repasser, tu transfert le toner sur le cuivre. Je dispose aussi d'une insoleuse (pro, double face, avec pompe a vide etc), et je ne l'utilise plus du tout, je trouve le transfer plus simple (par contre, pour faire du double face ca devient très compliqué voir impossible). J'ai aussi arrêté d'utiliser la méthode photo parceque l'insoleuse n'est pas chez moi (mais chez mes parents, ca prends de la place), et que j'ai toujours eu des soucis que ce soit avec le typon, à la révélation ou a la gravure (la température d'utilisation de tout ces produits est délicate). Et j'ai toujours eu l'impression que la graveuse, notament en chauffant le perchlo, avait tendance a faire partir la résine sensé protégé le cuivre. Pour la midibox, j'ai réaliser certain CI moi meme, mais dernièrement j'en ai commandé beaucoup chez Smash (http://www.midibox-shop.com/buy_lvo.html). Les prix sont bas, surtout pour la qualité du CI (double face, trou métalisé, sérigraphie), c'est beaucoup plus agreable à souder et solide. (le liens que je donne va directement sur la page pour les européens, avec des frais de port relativement bas, a partir du moment ou le montant total ne dépasse pas 22euros). Pour la sérigraphie sur les CI, tu peux le faire avec le transfer de toner (ca sera noir par contre, et pas blanc). Donc pour conclure : quand je peux acheter le CI (et a prix raisonable), je l'achete (ca a aussi l'avantage de faire vivre la communauté), sinon le grave moi meme (toner transfer et petit bac a perchlo, je fais aussi l'étamage avec de la pate à brasser et un décapeur). POur la sérigraphie sur les boitier, c'est plus compliqué : feuille autocollante imprimé, lettre transfert, pochoir (silk screen en anglais, la meilleur solution je pense, mais y'a un investissement de base), et pourquoi pas le toner transfer (mais pareil que pour les pcb, ca sera noir). Voila j'espere avoir répondu a tes questions! Mais j'en ai une aussi pour toi :) Tu dis que durant ton adolescence tu as construit une insoleuse, et il se trouve que pas plus tard que ce week end, j'ai voulus en réaliser une (pour cuire le vernis de protection des CI, et je veux pouvoir prendre le temps d'essayer différentes méthode et ne pas tacher mon insoleuse a CI pour ca). J'ais des tubes, starters et ballasts (récupérés au fil des années, la personne qui me les as donné les avait acheté pour faire une insoleuse), mais le soucis, c'est que les supports des tubes sont fait pour etre encastré (je suppose) dans une plaque d'acier fine, et que du coup, je n'ai aucune idée pour les monter correctement sur du bois (qui me semble le plus simple à assembler). Avec un peu de chance tu avais acheté les meme supports (qui semble etre classique, les miens datent des année 80 aussi, et on les trouve encore en vente chez gotronic par exemple), et que tu as trouvé le moyen de les monter?
-
Ok! So here's the DAC (sch + pcb). As I said, keep in mind that it maybe not perfect! (for example, on the schematic the DEM pin is connected to +5V, but in most application you want DEM off, so it needs to be wired to GND, on the PCB there's a pad to wire it to 5V or GND). It needs a +12V/-12V +5V analog power supply and a +5V digital supply (analog and digital ground are NOT connected on the PCB, they should be at some point, I did this on my PSU pcb). edit : mm it seems I can't attached sch and brd file in this post
-
Yes, this is for a full ADAT 8 in/8 out. The AL1201 DAC was made by Alesis-semi, they also made the AL1101 (ADC) and AL1401/AL1402 adat encoding/decoding cheap. Adn they provide schematic for this too. As I said in my other post, those cheap are now obselete, you can still find datasheet here : http://www.wavefrontsemi.com/index.php?products you can still find them at http://www.pcm63.com (and they are cheap). Of course I can share my schematic and PCB (but keep in mind that I'm no expert in PCB, and even the schematic design is not so good). Also, this is the same design as the Berhinger ADA8000 (minus the input preamp), which is VERY cheap (I started this project before Berhinger did this unit). The interesting things in my design is that you can use other DAC or ADC than the AL1201 or AL1101, use something elese for adat encoding/decoding (FPGA) as it's modular. I don't know where I can upload those and put some information, some kind of a blog maybe?
-
Yes I need to find a place to put them back on line. As you find, it started on an old thread on this forum, 10 years ago... I get back the project recently (it was working, but I didn't do anything right the first time, so now I'm finishing it in a better way).
-
I use this DAC for an ADAT converter: http://www.pcm63.com/?222,al1201 It's cheap and comes in a SOIC package (easy to solder). It can works with I2S (actually it doesn't require master and bitclock, only LR clock (48Khz) and data). The only drawbacks are it can do only 48Khz max, and its now an obselete chip. I've got eagle schematic + board (single sided) if you're interested.
-
On peut commander en "petit" paquet, pour passer outre la douane, pour les CI uniquement. C'est très peu cher, surtout vu la qualité des CI. Tim fait beaucoup pour la communauté, et c'est effectivement quelqu'un de très sérieux et très amical.
-
Le fader dans la video est capable de se positioner parcequ'il a une piste "servo" qui permet de connaitre sa position (exactement comme les faders utilisables avec le module MBMF). Controller un potentiomètre, qui a une piste servo, c'est pas difficile. Ce qui risque de l'etre un peu plus, c'est de trouver des potentiomètre pour ton ampli (c'est a dire les bonnes valeurs et les bonnes courbes), motorisé et avec piste servo (et si tu trouve, ca risque de couter un bras). Il existe des solution alternative au potentiomèter numérique. Avec des relais, tu peux simuler un potentiomèe (avec 8 relais et 16 resistances, tu peux faire un potentiomètre totalement analogique avec une resolution de 8bits, mais si tu as besoin d'avoir une variation log, tu n'aura pas beaucoup de valeur). Tu peux aussi essaye avec des LDR illuminé par une led dont tu fait varier l'intensité. Peut etre avec des FET aussi.